An Impeccable Lesson Plan — 22 chapters and 340 lessonsYour Step-By-Step Guide To JavaScript Mastery
We’ve crafted an impeccable lesson plan that helps you go from beginner to senior in less than 6 months (should you dedicate sufficient time and energy to go through the course).
This lesson plan guides you through every step of the learning—showing you everything you need to know, including coding patterns, best practices, and even accessibility.
(6 months is a short time for a huge topic like JavaScript—many developers have spent years learning JavaScript and they’re still not as good as you’ll be in 6 months).
Chapter 1JavaScript and its ecosystem
Put the concepts and ideas in the right places by first understanding the ecosystem.
- Welcome to Learn JavaScript!
- What is JavaScript used for?
- The JavaScript ecosystem
- Varying versions of JavaScript
Chapter 2JS Basics
Get to know the foundational basics where you'll build the rest of your skills on.
Learn JavaScript is my number one choice for learning JavaScript. I finally feel that JavaScript is within my reach.
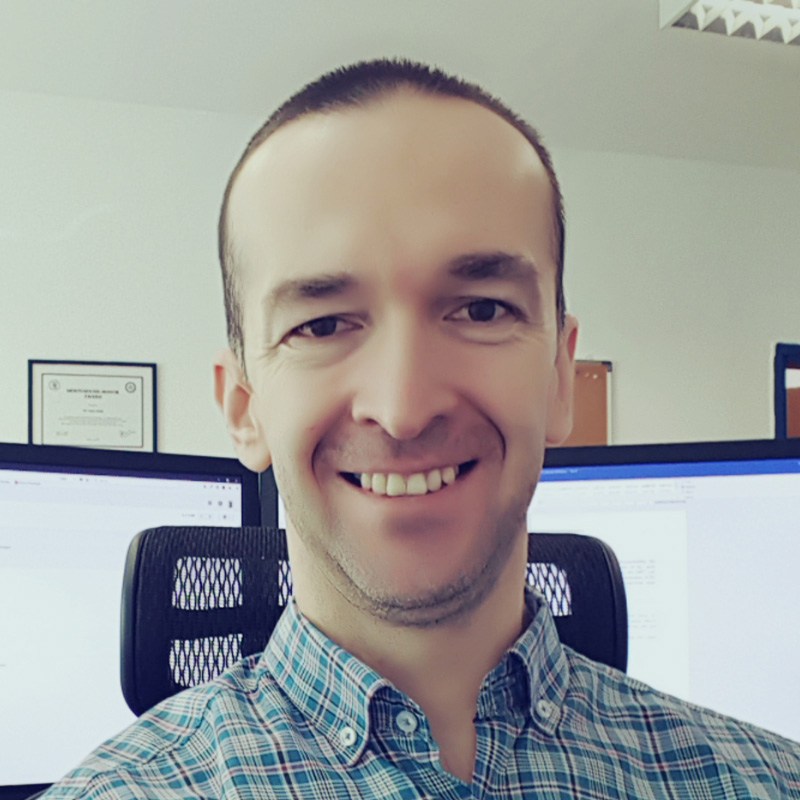
- Linking your JavaScript file
- Preparing your text editor
- The Console
- Comments
- On semicolons
- Strings, numbers and booleans
- Understanding Variables
- Understanding Functions
- The Flow of a Function
- Arrow functions
- Intro to objects
- If/else statements
- Comparing Objects
- The NOT operator
- Null and Undefined
- The BOM and the DOM
- Selecting an Element
- Changing Classes
- Listening to events
- Callbacks
Chapter 3Building simple components
Begin to think like a developer by building simple components. 🛠 signifies practical lessons where I show you how to build things.
- How to think like a developer
- Starter files and Source codes
- Do this for every component
- 🛠 Off-canvas menu: Building an off-canvas menu
- 🛠 Modal: Building a Modal
- Lessons from the building process
- Debugging errors
- How to use a linter
Chapter 4Arrays and loops
Gain clarity over the most confusing chapter for newbies.
- Introduction to Arrays
- Array methods
- For loops
- The forEach loop
- Selecting multiple elements
- Nodes vs Elements
- 🛠 Accordion: Building an accordion
Chapter 5Dom basics
Have full control over the DOM — and make magic happen.
Learn JavaScript will turn you into a JavaScript wizard. It is one of my favorite resources due to Zell’s ability to present content in a crystal clear manner.
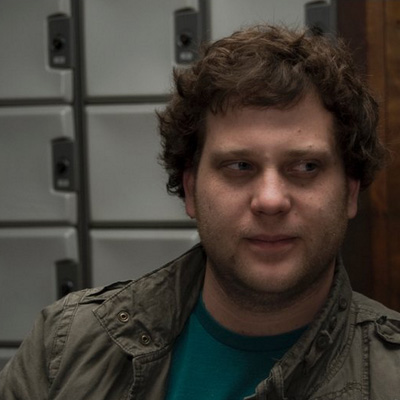
- Id, classes, attributes, and tags
- Changing CSS with JavaScript
- Getting CSS with JavaScript
- Changing Attributes
- Finding an element's size and position
- DOM Traversals
- 🛠 Tabby: Building Tabby (A Tabbed component)
- 🛠 Carousel: HTML and CSS
- 🛠 Carousel: Switching slides with JavaScript
- 🛠 Carousel: Working the dots
- 🛠 Carousel: Positioning slides with JavaScript
Chapter 6Events deep dive
Gain mastery over Events, which is a core system in Vanilla JavaScript.
- The listening element
- Default Behaviors
- Event propagation
- Event delegation
- Removing Event Listeners
- 🛠 Modal: Closing the modal
- 🛠 Accordion: Event delegation
- 🛠 Tabby: Event delegation
- 🛠 Carousel: Event delegation
Chapter 7Transitions and Animations
Learn to create smooth and buttery animations that enhances everything you build.
I love the step-by-step structure in Learn JavaScript. I also love the text-based approach to lessons. They helped me understand and internalise JavaScript instead of feeling “I just watched some videos”.
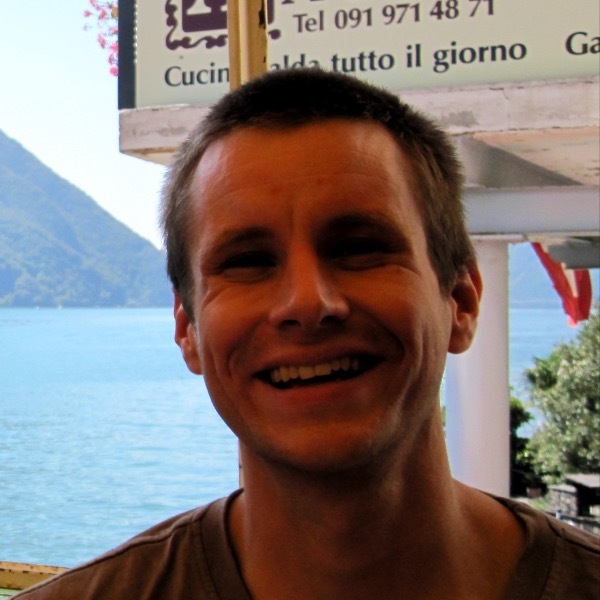
- CSS Transitions
- CSS Animations
- Silky-smooth animations
- Integrating CSS transitions and animations with JavaScript
- Animating with JavaScript
- GreenSock Animation API (GSAP)
- 🛠 Off-canvas menu: Animations
- 🛠 Modal: Animating the modal
- 🛠 Modal: Animating the pointing hand
- 🛠 Modal: Animating the waving hand
- 🛠 Modal: Wave hand animation with JavaScript (using GSAP)
- 🛠 Accordion: Animations
- 🛠 Carousel: Animations
Chapter 8Useful JS features
Use the best JavaScript features to make coding much easier
- Ternary operators
- AND and OR operators
- Early returns
- Template Literals
- Destructuring
- Default parameters
- Enhanced Object Literals
- Rest and Spread
- Useful array methods
- Reduce
- Looping through objects
- Returning objects with implicit return
- 🛠 Accordion: Using useful JavaScript features
- 🛠 Tabby: Using useful JavaScript features
- 🛠 Carousel: Useful JavaScript features
Chapter 9JS Best practices
Use industry-proven best practices to write code that's easy to read, maintain, and debug
This is the first course where I learned to differentiate which parts of JavaScript are important and which parts are not.
I used the knowledge I learned to create real components. It’s very satisfying to see my code doing something of real and practical use.
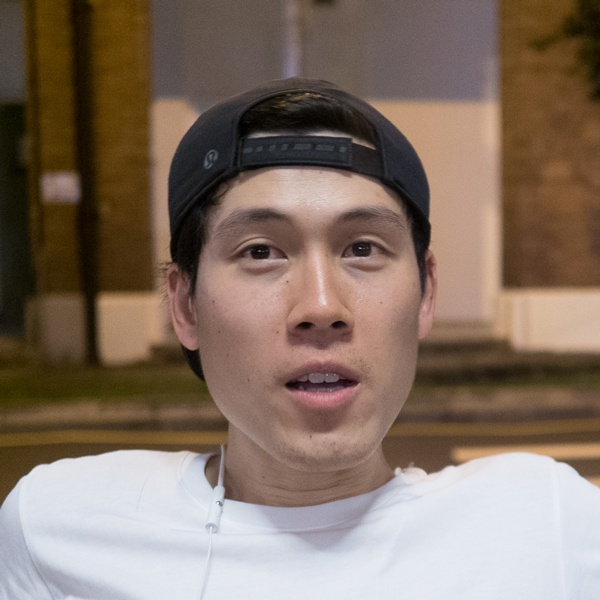
- Write declarative code
- Functions with a purpose
- Manage scope
- Reduce state changes
- Don't reassign
- Don't mutate
- Preventing Objects from mutating
- Preventing Arrays from mutating
- Write pure functions
- 🛠 Accordion: Refactor
- 🛠 Carousel: First refactor
- 🛠 Carousel: Refactoring the dots part
- 🛠 Carousel: Previous and next buttons
- 🛠 Carousel: Second refactor
Chapter 10Manipulating text and content
More DOM goodness — which means more power and magic over what you can do.
I wish Learn JavaScript was around when I first tried to learn JavaScript. It changed the way I approach JavaScript. Now, I actually know what I’m doing when I write JavaScript!
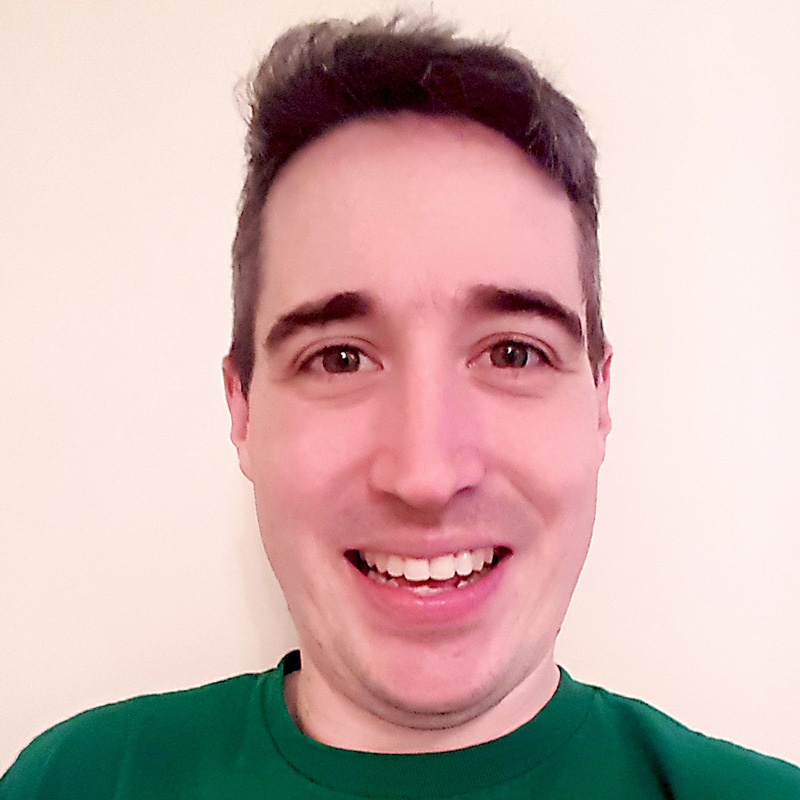
- Changing Text and HTML
- Creating HTML Elements
- Adding multiple elements to the DOM
- Removing Elements from the DOM
- 🛠 Carousel: Creating dots with JavaScript
- 🛠️ Calculator: HTML and CSS
- 🛠️ Calculator: Happy Path
- 🛠️ Calculator: Testing the Happy Path
- 🛠️ Calculator: Easy Edge Cases
- 🛠️ Calculator: Difficult Edge Cases
- 🛠️ Calculator: Refactoring
- The switch statement
- 🛠️ Calculator: Refactoring (Part 2)
- 🛠️ Popover: Making one popover
- 🛠️ Popover: Making four popovers
- 🛠️ Popover: Making popovers with JavaScript
Chapter 11Handling Forms
Everything you need to know about forms to build websites and apps
- Intro to forms
- Selecting form fields with JavaScript
- Form fields and their events
- Sanitize your output
- Generating unique IDs
- 🛠️ Popover: Dynamic ID
- 🛠️ Todolist: The HTML and CSS
- 🛠️ Todolist: Creating tasks with JavaScript
- 🛠️ Todolist: Deleting tasks with JavaScript
- 🛠️ Typeahead: The HTML and CSS
- 🛠️ Typeahead: Displaying predictions
- 🛠️ Typeahead: Selecting a prediction
- 🛠️ Typeahead: Bolding search terms
Chapter 12Handling Dates
Everything you need to know about Date and Time in JavaScript—without using any libraries.
Learn JavaScript is an incredible resource that helped me save time from searching around the internet for answers! It showed me the full landscape of the Vanilla JavaScript ecosystem.
Every time I jump into the course, I deepen my javascript knowledge and I become more marketable for work in the future.
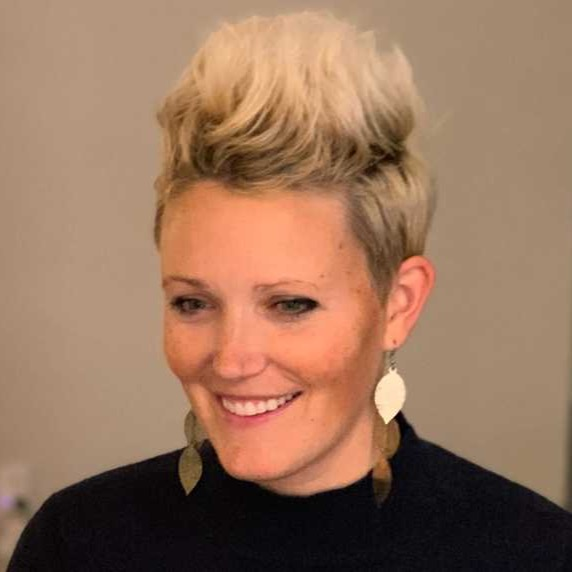
- The Date object
- Getting a formatted date
- Getting the time
- Local time and UTC Time
- Setting a specific date
- Setting a date with Date methods
- Adding (or subtracting) date and time
- Comparing Dates and times
- 🛠️ Datepicker: HTML and CSS
- 🛠️ Datepicker: Building the calendar
- 🛠️ Datepicker: Building the datepicker with JavaScript
- 🛠️ Datepicker: Previous and Next buttons
- 🛠️ Datepicker: Selecting a date
- 🛠️ Datepicker: Positioning the datepicker
- 🛠️ Datepicker: Showing and hiding
- Formatting a date with toLocaleString
- setTimeout
- setInterval
- 🛠️ Countdown timer: HTML and CSS
- 🛠️ Countdown timer: JavaScript
- 🛠️ Countdown timer: Counting Months
- 🛠️ Countdown timer: Daylight Saving Time
- 🛠️ Countdown timer: Counting Years
Chapter 13Async JS
Master almost everything you need about Async JS—except building servers for Rest APIs (which is covered in another course).
Zell is the rare sort of developer who both knows his stuff and can explain even the most technical jargon in approachable — and even fun! — ways.
I’ve taken his courses and always look forward to his writing because I know I’ll walk away with something that makes me a better front-ender.
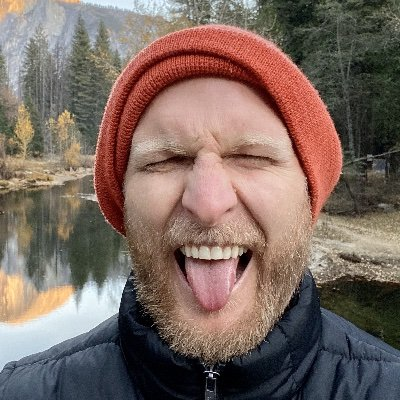
- Introduction to Ajax
- Understanding JSON
- The Fetch API
- Possible data types
- JavaScript Promises
- Requests and responses
- Sending a POST request
- Authentication
- Handling errors
- Viewing response headers
- CORS and JSONP
- XHR vs Fetch
- Using an Ajax library
- Reading API documentation
- Understanding curl
- 🛠️ Todolist: The Todolist API
- 🛠️ Todolist: Fetching tasks
- 🛠️ Todolist: Creating tasks
- 🛠️ Todolist: Editing tasks
- 🛠️ Todolist: Deleting tasks
- 🛠️ Todolist: Creating tasks with Optimistic UI
- 🛠️ Todolist: Handling Optimistic UI errors
- 🛠️ Todolist: Editing tasks with Optimistic UI
- 🛠️ Todolist: Deleting tasks with Optimistic UI
- 🛠️ Todolist: Refactor
- 🛠️ Typeahead: How to add Ajax
- 🛠️ Typeahead: Adding Ajax
- 🛠️ Typeahead: Handling errors
- 🛠️ Google Maps Clone: Creating your first Google Map
- 🛠️ Google Maps Clone: Fetching JSONP via JavaScript
- 🛠️ Google Maps Clone: Drawing directions
- 🛠️ Google Maps Clone: Driving directions
- 🛠️ Google Maps Clone: Handling errors
- 🛠️ Google Maps Clone: Adding stopovers
- 🛠️ Google Maps Clone: Refactor
- Requesting many resources at once
- Asynchronous functions
- Handling multiple awaits
- Asynchronous loops
- 🛠️ Dota Heroes: Listing heroes
- 🛠️ Dota Heroes: Filtering heroes (Part 1)
- 🛠️ Dota Heroes: Filtering heroes (Part 2)
- 🛠️ Dota Heroes: Refactoring
- 🛠️ Dota Heroes: Hero Page
- 🛠️ Dota Heroes: Making the hero page robust
- 🛠️ Dota Heroes: Heroes page refactor
Chapter 14Advanced Async JS
Real and practical stuff you'll need to do, beyond the basics.
- Requesting many resources at once
- Asynchronous functions
- Handling multiple awaits
- Asynchronous loops
- 🛠️ Dota Heroes: Listing heroes
- 🛠️ Dota Heroes: Filtering heroes (Part 1)
- 🛠️ Dota Heroes: Filtering heroes (Part 2)
- 🛠️ Dota Heroes: Refactoring
- 🛠️ Dota Heroes: Hero Page
- 🛠️ Dota Heroes: Making the hero page robust
- 🛠️ Dota Heroes: Heroes page refactor
Chapter 15Handling Keyboard Events
Make your website and app keyboard accessible, and give power users keyboard shortcuts to play with.
Learn JavaScript gave me a deep understanding of the language and its capabilities. Even though I am a professional web developer, I gained new perspectives on things I already know, and I also learned new things that I didn’t know before.
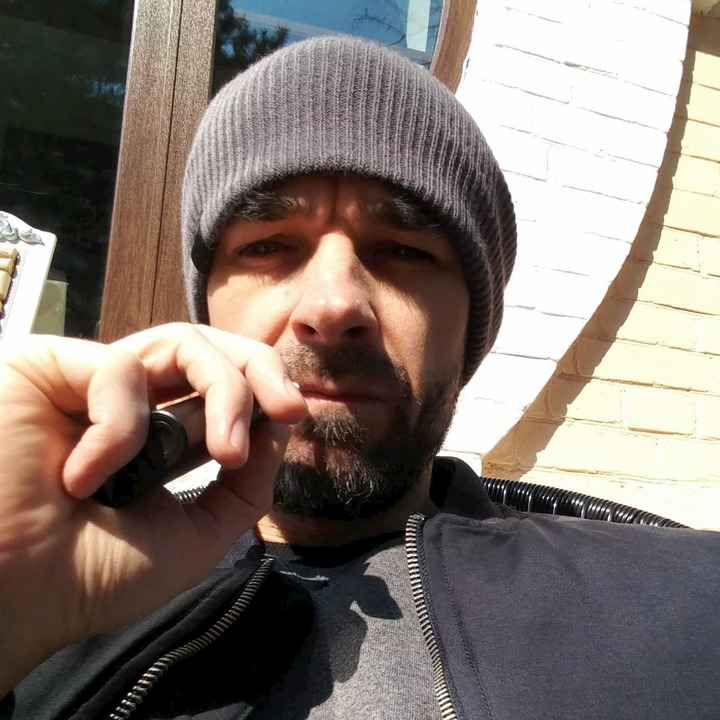
- Keyboard users
- Handling commonly used keys
- Keyboard events
- Understanding Tabindex
- Detecting the focused element
- Directing focus
- Preventing people from tabbing into elements
- How to choose keyboard shortcuts
- Creating single-key shortcuts
- 🛠️ Off-canvas: Adding keyboard interaction
- 🛠️ Modal: Adding keyboard interaction
- 🛠️ Accordion: Adding keyboard interaction
- 🛠️ Tabby: Adding keyboard interaction
- 🛠️ Tabby: Refactoring
- 🛠️ Carousel: Adding keyboard interaction
- 🛠️ Carousel: Displaying help text
- 🛠️ Calculator: Adding keyboard interaction
- 🛠️ Popover: Keyboard
- 🛠️ Popover: Refactor
- Keyboard shortcuts with Command and Control modifiers
- 🛠️ Todolist: Keyboard
- 🛠️ Typeahead: Keyboard
- 🛠️ Typeahead: Selecting a prediction with the keyboard
- 🛠️ Google Maps Clone: Keyboard
- 🛠️ Dota Heroes: Keyboard
- 🛠️ Datepicker: Tabbing in and out
- 🛠️ Datepicker: Keyboard shortcuts
Chapter 16Screen reader accessibility
Everything you need to know about JavaScript accessibilty written for self-taught developers.
I went from knowing nothing about JavaScript to building a custom design system for my company entirely in JavaScript. What’s more, each component I build is also accessible thanks to Zell’s accessibility lessons
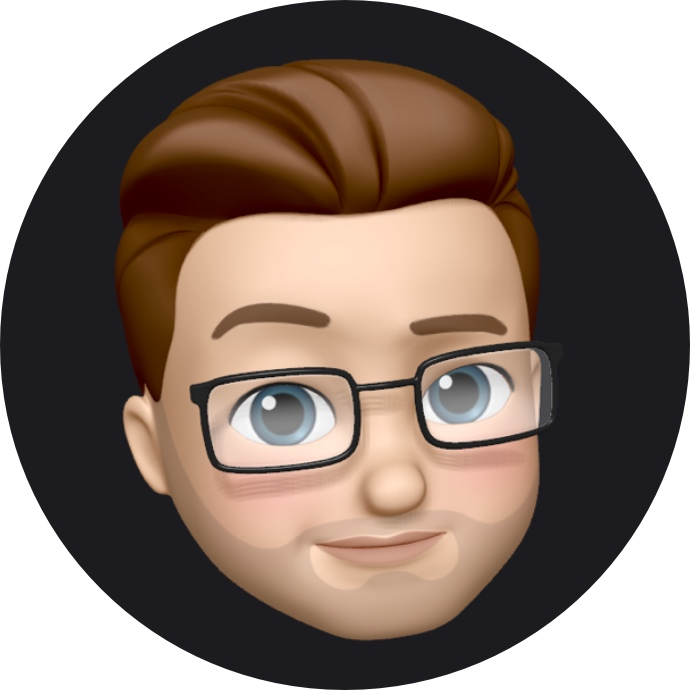
- What is accessibility?
- How to use a screen reader
- Using NVDA
- Using Voiceover
- Aria roles
- Landmark roles
- Document structure roles
- Live region roles
- Widget roles
- Window and Abstract roles
- Accessible names and descriptions
- Hiding content
- ARIA properties and ARIA states
- ARIA for expandable widgets
- 🛠️ Off-canvas: Accessibility
- ARIA for modal dialogs
- 🛠️ Modal: Screen reader accessibility
- 🛠️ Accordion: Screen reader accessibility
- ARIA for Tabbed components
- 🛠️ Tabby: Screen reader accessibility
- 🛠️ Tabby: Refactor
- 🛠️ Carousel: Screen reader accessibility
- Roles that trigger Forms and Application modes
- What's next for accessibility?
Chapter 17Handling Scroll
Learn to add fancy animations that gives your website the zing when a user scrolls.
- The Scroll event
- 🛠️ Auto-hiding Sticky-nav: HTML and CSS
- 🛠️ Auto-hiding Sticky-nav: JavaScript
- 🛠️ Auto-hiding Sticky-nav: Natural reveal
- Intersection Observer API
- Intersection Observer Options
- 🛠️ Slide & Reveal
- 🛠️ Slide & Reveal: Always fade-in when you scroll down
- 🛠️ Slide & Reveal: Fine-tuning the animation
- 🛠️ Infinite Scroll: Anatomy
- 🛠️ Infinite Scroll: Infinite load
- 🛠️ Infinite Scroll: Refactor
- 🛠️ Infinite Scroll: Implementing the Infinite Scroll
Chapter 18Mouse, Touch, and Pointer events
Everything you need to know about handling mouse, touch, and pointers.
- Mouse Events
- 🛠️ Spinning Pacman: HTML and CSS
- 🛠️ Spinning Pacman: JavaScript
- Touch events
- Pointer events
- Touch-action
- 🛠️ Spinning Pacman: Supporting Touch
- Cloning elements
- 🛠️ DragDrop: HTML and CSS
- 🛠️ DragDrop: JavaScript
- 🛠️ DragDrop: Creating a drop preview
- 🛠️ DragDrop: Sortable drop preview
- 🛠️ DragDrop: Robustness
- 🛠️ DragDrop: Refactor
Chapter 19Object Oriented Programming
Learn about this major JavaScript pillar. We only cover this here because you need sufficient skill before diving into this chapter.
Learn JavaScript helped me discover holes in my knowledge even though I have a few years of JavaScript experience!
I became more confident when working with frameworks and libraries, and I spend less time on Google and Stack Overflow.
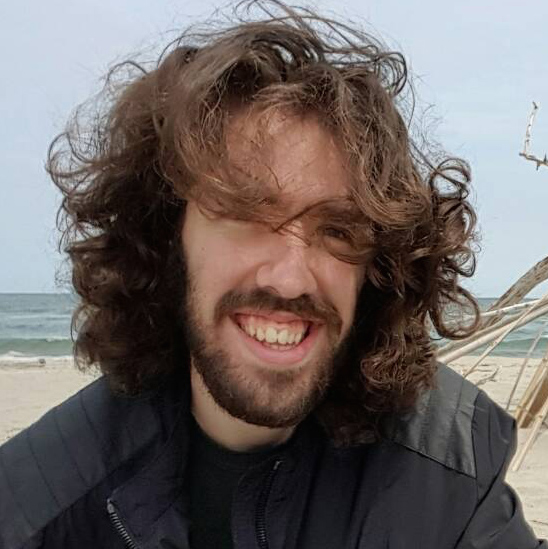
- Introduction to Arrays
- Array methods
- For loops
- The forEach loop
- Selecting multiple elements
- Nodes vs Elements
- 🛠 Accordion: Building an accordion
- Before we begin
- What is Object Oriented Programming?
- Four Flavours of Object Oriented Programming
- Inheritance
- This in JavaScript
- Call, bind, apply
- Creating Derivative Objects
- Composition vs Inheritance
- Polymorphism
- Encapsulation
- Closures
- Encapsulation in Object Oriented Programming
- Getters and Setters
- What OOP flavour to use
- When to use Object Oriented Programming
Chapter 20Writing reusable code
Learn to make your code reusable so you can build stuff faster.
Learn JavaScript is excellent. It is beginner friendly and has very clear explanations. It does not assume I knew anything and zip through things unlike other courses.
It’s very easy for me to learn with the examples and source code provided.
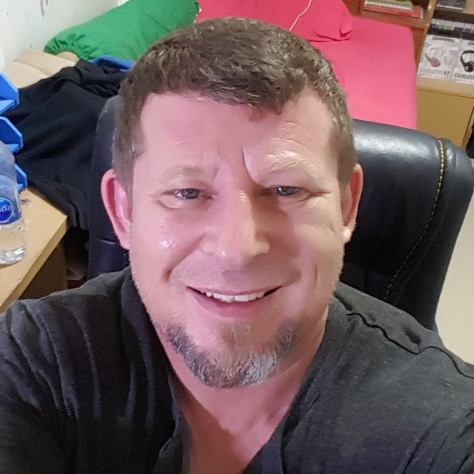
- Creating reusable code by writing libraries
- Two Types of libraries
- Including libraries with Script tags
- Including libraries with ES6 Modules
- Dynamic imports
- 🛠️ Off Canvas: Building a Library
- 🛠️ Modal: Library setup
- 🛠️ Modal: Opening the Modal
- 🛠️ Modal: Closing the modal
- 🛠️ Modal: Inheritance and Polymorphism
- 🛠️ Modal: Resolving differences between subclasses
- 🛠️ Modal: Exposing properties and methods
- 🛠️ Accordion: Building a library
- 🛠️ Tabby: Building a library
- 🛠️ Carousel: Building a library
- 🛠️ Calculator: Library
- 🛠️ Calculator: Fixing the Clear Key
- 🛠️ Calculator: Handling other keys
- 🛠️ Calculator: State
- 🛠️ Popover: Library
- 🛠️ Popover: Adding event listeners
- 🛠️ Typeahead: Library
- 🛠️ DatePicker: Library
Chapter 21Going from Vanilla JS to JS Frameworks
Understand how to use a framework by building one — absolutely overkill, but you emerge with incredibly deep skill and expertise
- 🛠️ Building a Tiny framework
- 🛠️ Tiny: Add event listeners
- 🛠️ Tiny: Updating state
- 🛠️ Tiny: Rendering Child Components
- 🛠️ Tiny: Changing Parent State
- 🛠️ Tiny: Passing Props
- 🛠️ Tiny: Multiple Props
- 🛠️ Tiny: Passing values from sibling components
- 🛠️ Tiny: Mounting
- 🛠️ Tiny: Passing props to descendants
- 🛠️ Tiny: A tiny refactor
Chapter 22Building Single Page Apps
Learn everything you need about building Single Page Apps without the use of a framework — another overkill, but you understand what all framework routers do.
This is the course I’ve been needing my whole career. If you really want to learn JavaScript, you need this course.
I now feel so much more confident in JavaScript. I can read and understand JavaScript without relying on Google for answers.
And I love having Zell as a mentor. He is patient and willing to teach me and other members of the community
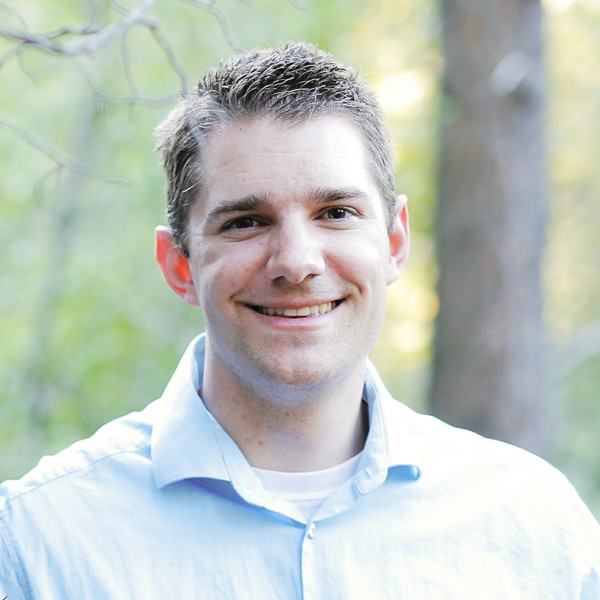
- What is a Single Page App?
- Simple SPA using only CSS
- The Location Interface
- The History Interface
- Minimum viable server for a SPA
- 🛠️ Dota SPA: Introduction
- 🛠️ Dota SPA: Building The Heroes List
- 🛠️ Dota SPA: Building the filters
- 🛠️ Dota SPA: Filtering heroes
- 🛠️ Dota SPA: Displaying filtered heroes
- 🛠️ Dota SPA: Getting Ready to build the Hero Page
- 🛠️ Dota SPA: Building the hero page
- 🛠️ Dota SPA: Lore and abilities
- 🛠️ Dota SPA: Routing for Single-page apps
Trusted by Industry Professionals and Publications
I wish Learn JavaScript was around when I first tried to learn JavaScript. It changed the way I approach JavaScript. Now, I actually know what I’m doing when I write JavaScript!
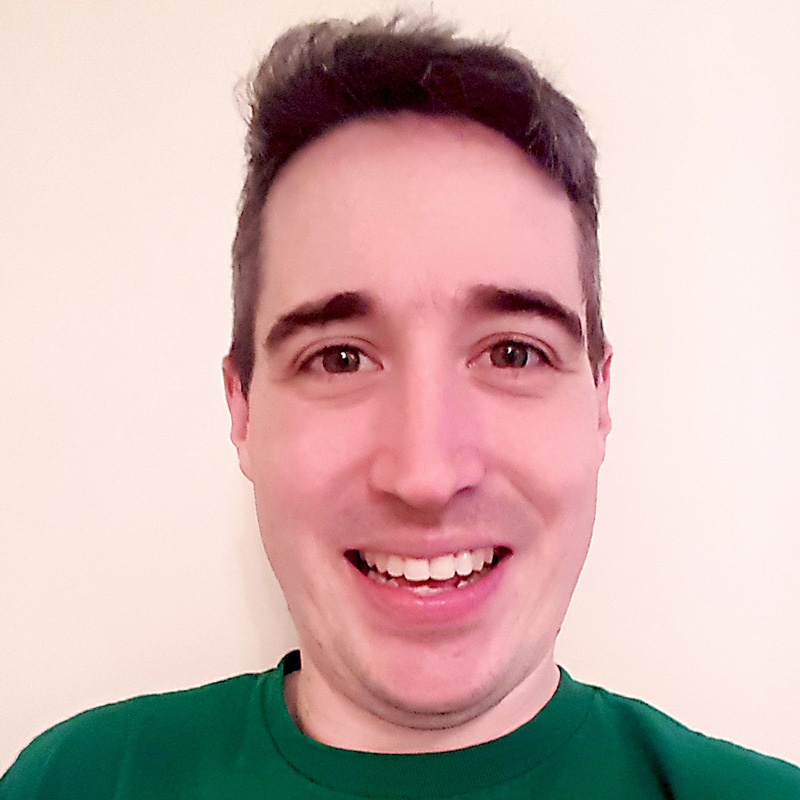
Zell is the rare sort of developer who both knows his stuff and can explain even the most technical jargon in approachable — and even fun! — ways.
I’ve taken his courses and always look forward to his writing because I know I’ll walk away with something that makes me a better front-ender.
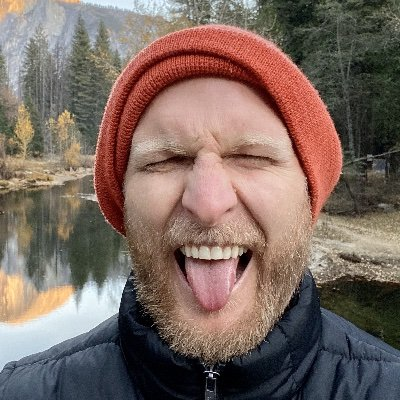
Your articles are honestly the best resources out there! They have really helped 100Devs folx understand topics that are always stumbling blocks for new devs.
Really appreciate the work you put into your content!
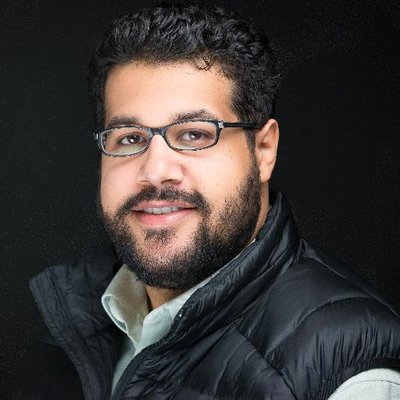
If you’re looking to learn JavaScript, Zell just made a new course that shows how to build 20 real components from scratch, step by step.
Zell is a really fun person too, so that helps when you’re trying to learn :)
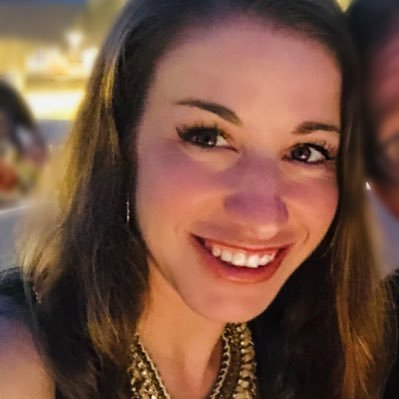
Zell is one of those rare people who commands tremendous knowledge and experience but remains humble and helpful.
They want you to know what they know, not just be impressed by it. In other words, Zell is a natural teacher. You’re lucky to have him because he feels lucky to be able to help you in your journey.
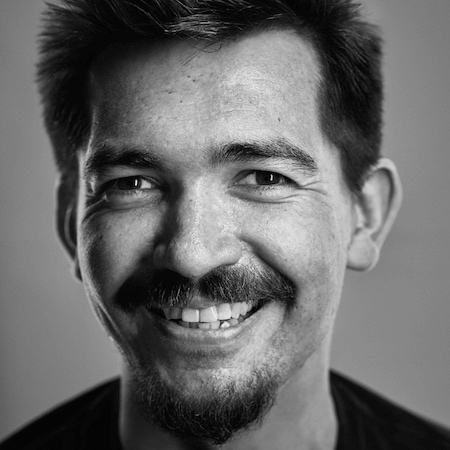
Zell has a really comprehensive style so I’m stoked to have him dishing out some Gulp on CSS Tricks.
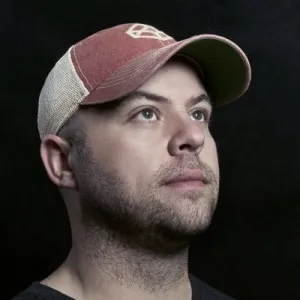
There’s a right plan for everyoneIt’s Time For Your To Become An Amazing JavaScript Developer
Just select a plan to begin. Before you know it, you’ll be able to build anything you want with JavaScript.
Adept$275
(Or 6 payments of $55)
Mastery$495
(Or 6 payments of $99)
Everything$50/m
(Save 2 months with annual billing)